11-vuex数据仓库
Vuex 是一个专为 Vue.js 应用程序开发的(数据的)状态管理模式。
Vuex采用“集中式存储管理”方式去管理数据,把 这些数据 应用到 所有组件上 !!!
vuex就是仓库---数据仓库--这个数据仓库里的所有的数据,是为组件来使用的!为组件来服务的!
vuex 只 做 “数据” 的维护!!
对vuex仓库中数据的处理,我们都放在“模型”里面。在vuex这个仓库 store 里面。
vuejs官网
https://cn.vuejs.org/
vuejs--生态系统--核心插件
https://vuex.vuejs.org/zh/installation.html
一、vuex下载和安装和使用 --------------------------------------------------------------
下载
(1)使用script标签引入
直接引用
https://unpkg.com/vuex@3.6.2/dist/vuex.js
下载本地,引用
<script src="/js/vue.js"></script>
<script src="/js/vuex.js"></script>
(2)安装vuex
npm install vuex --save
(3)使用vuex
安装后,在工程化项目中,使用Vuex插件之前需要在main.js入口文件中
a.使用import方式引入Vuex
import Vuex from 'vuex'
b.使用Vuex的插件引入函数Vue.use()使用Vuex
Vue.use(Vuex);
(4)创建数据仓库
创建数据仓库
var store = new Vuex.Store({})
例如:创建数据仓库(存储数据) var store = new Vuex.Store({ // 存数据 state:{ totalPrice:10000, goods:[ { id:1, goodname:'戴尔笔记本', price:3999, num:30 }, { id:2, goodname:'惠普打印机', price:1560, num:10 }, { id:3, goodname:'戴尔台式电脑', price:4580, num:100 } ] } });
(5)引入到组件(因为,引入到根组件之后,所有的子组件,都可以直接用store数据仓库里面的数据) store:store, 或 store,
var vm = new Vue({
el: '#app',
// 引入到组件 store:store, 或 store,
store,
data: {
},
methods: {
},
components: {
Child
}
});
vuex核心概念
state 存储数据
getters 获取数据的方式
mutations 修改数据的方式
actions 通过后台更改操作异步数据的方式
以上,这几个 都是对象
// 如果希望模块不是全局的,我们在这里 需要添加命名空间
namespaced:true,
state:{},
getters:{},
mutations:{},
actions:{}
当我们不申明命名空间的时候
state:{}, 这个方法是局域的
getters:{}, 其他的几个方法是全局的
mutations:{},
actions:{}
二、下面首先看 state的使用
1.数据仓库store 定义好公共数据 --------------------------------------------------------------
var store = new Vuex.Store({
// 存数据
state:{
totalPrice:10000,
goods:[
{
id:1,
goodname:'戴尔笔记本',
price:3999,
num:30
},
{
id:2,
goodname:'惠普打印机',
price:1560,
num:10
},
{
id:3,
goodname:'戴尔台式电脑',
price:4580,
num:100
}
]
}
});
2.组件 访问数据仓库store 中的公共数据,组件 必须 使用 计算属性 computed---------------------------------------
我们在 计算属性中 使用 this.$store.state.xxx 获取 数据仓库中state中的数据
// 子实例(子组件)
var Child = {
template:'#child',
// 计算属性
computed:{
totalPrice:function(){
return this.$store.state.totalPrice
},
goods:function(){
return this.$store.state.goods
}
}
}
computed计算属性的核心就是计算!!!
什么时候计算?里面用到的变量,发生变化,它就进行计算。
computed计算属性的特点:监听,当computed里面的值发生变化的时候,
数据仓库中的state中的totalPrice 会跟着变化!!
所以,我们要使用“计算属性”来从“仓库”里面拿“数据”。
3.组件 通过 计算属性 computed 拿到 数据仓库 数据,将数据 显示在 该组件的 视图层---------------------------------------
<template id="child">
<div>
<p>我是child组件</p>
<p>总价:{{totalPrice}}</p>
<table width="600" border="1">
<tr>
<th>商品编号</th>
<th>商品名称</th>
<th>商品单价</th>
<th>商品数量</th>
<th>商品小计</th>
</tr>
<tr v-for="(item,index) in goods">
<td>{{item.id}}</td>
<td>{{item.goodname}}</td>
<td>{{item.price}}</td>
<td>{{item.num}}</td>
<td></td>
</tr>
</table>
</div>
</template>
<script src="./js/vue.js"></script> <!-- 一.引入vuex --> <script src="./js/vuex.js"></script> </head> <body> <div id="app"> <child></child> </div> <template id="child"> <div> <p>我是child组件</p> <p>总价:{{totalPrice}}</p> <table width="600" border="1"> <tr> <th>商品编号</th> <th>商品名称</th> <th>商品单价</th> <th>商品数量</th> <th>商品小计</th> </tr> <tr v-for="(item,index) in goods"> <td>{{item.id}}</td> <td>{{item.goodname}}</td> <td>{{item.price}}</td> <td>{{item.num}}</td> <td></td> </tr> </table> </div> </template> <script> // 子实例(子组件) var Child = { template:'#child', // 计算属性 computed:{ totalPrice:function(){ return this.$store.state.totalPrice }, goods:function(){ return this.$store.state.goods } } } // 二.使用Vuex的插件 引入函数Vue.use() Vue.use(Vuex) // 三、创建数据仓库(存储数据) var store = new Vuex.Store({ // 存数据 state:{ totalPrice:10000, goods:[ { id:1, goodname:'戴尔笔记本', price:3999, num:30 }, { id:2, goodname:'惠普打印机', price:1560, num:10 }, { id:3, goodname:'戴尔台式电脑', price:4580, num:100 } ] } }); // 根实例(根组件) var vm = new Vue({ el: '#app', // 四、引入到组件(因为,引入到根组件之后,所有的子组件,都可以直接用store数据仓库里面的数据) store:store, 或 store, store, data: { }, methods: { }, components: { Child } }); </script>
三、下面接着看 getters的使用
getters 获取仓库里面的数据 ------------------------------------------------------
获取数据的时候,如果 说 你 想在 获取的时候 对数据 进行 计算。
通过 计算 改 state中的数据的状态
(计算---你去做事情,你做的事情,写在哪里?写在方法里,或者 我们说 写在函数里)
(使用getters,它就有逻辑了,它就能思考了。)
getters:{
下面这3种写法 均可。
totalPrice:state=>{
},
totalPrice:function(state){
},
totalPrice(state){
}
}
例如1.使用getters计算“每一件商品”的总价
// 获取仓库里面state上的数据,并且 对数据 进行了 计算 getters:{ // 1.使用getters计算“每一件商品”的总价 // 思路:在商量列表goods这个数组里,每一条数据中,添加一个数据项,定义名称total,并且把这个数据项total放置到 每一项数据中 getGoods:function(state){ // 遍历数组 let goods = state.goods; goods.forEach((item)=>{ // 注意:total这个属性,是我们在遍历的过程中,人为的 往商品身上 添加的一个属性 item.total = item.price*item.num }) return goods; } }
例如2.使用getters计算所有商品总价
// 2.使用getters计算所有商品总价 getTotalPrice:(state)=>{ let totalPrice = state.totalPrice; let goods = state.goods; // 遍历数组 goods.forEach((v)=>{ totalPrice += v.price*v.num }) return totalPrice; }
组件中
// 子实例(子组件) var Child = { template:'#child', // 计算属性 computed:{ totalPrice:function(){ return this.$store.getters.getTotalPrice }, goods:function(){ return this.$store.getters.getGoods } } }
组件的视图层中
<p>所有商品的总价:{{totalPrice}}</p>
四、下面接着看 mutations的使用
mutations 修改仓库里面的数据 ---------------------------
mutations 这个是修改仓库里的数据。(同步修改)
我们使用vuex数据仓库中的state和getters 获取数据,但是 现在唯独不能改这里的数据。我们是 用 mutations 改仓库中的数据!
在组件中,想要操作 仓库里面的数据,我们使用commit() “调用” mutations里面的方法
this.$store.commit('mutations里面的方法',值)
例如:
this.$store.commit('del',{id})
'del'---第一个参数 是 仓库里 mutations里面的 方法
{id} ---第二个参数 是 传递给 仓库里mutations方法里的 参数
vuex-----使用mutations(操作)修改 仓库 数据
(1) 我们要访问一个动作----移除
删除,传一个商品编号id
<button @click="del(v.id)">删除</button>
(2) 组件Lists写一个方法,写删除“传过来的一个id”
注意:这个组件里 没有 数据!这个数据在仓库里面,
所以 它的有个办法,能调用 仓里里的“动作”
组件中视图层
<button @click="del(item.id)">删除</button>
组件中方法中心
methods:{ del(id){ // 找到这个仓库,使用commit() “调用” mutations里面的方法 // 调用del删除,删除传入参数id this.$store.commit('del',{ id:id }) } } 注意: {id:id} 可以{id}
仓库中mutations方法中
// mutations 这个是修改仓库里的数据。(同步修改) mutations:{ // 使用mutations(操作)修改 仓库 数据 del:(state,param)=>{ console.log('仓库接收,组件传过来的值:----'+param); let k; // 遍历商品列表,在遍历的过程中,删 其中你点击的 那一条数据 let goods = state.goods; goods.forEach((v,i)=>{ // 在遍历的过程当前 去比对 // 我们在遍历的过程当中,如果组件传过来的 值 和 当前这个商品的id 相等,说明你点击 的就是这个商品!! if(param == v.id){ k = i } }) console.log('当前你点击这个商品的索引是:----'+k); // 从数组的 指定位置k 上,删1个内容 goods.splice(k,1); } }
预览效果,如下所示:
五、下面接着看actions的使用
actions 通过 后台更改操作 异步 修改 数据仓库state中的数据的
actions 这个异步操作
vuex-----使用actions与axios异步 初始化 页面中的商品列表上 的数据
1.需要引入axios。------------------
(1)引入script
<script src="./js/axios.js"></script>
(2)使用npm安装
npm install vuex --save
2.在state中goods里的数据,是通过后台来抓取。所以这里默认是 goods:[]空数组。------------------
state: { // 总价 totalPrice:100, goods:[] },
3.现在我们通过 在 php服务器端,模拟数据,并将数据转成json格式。------------------
<?php $data = [ ['id'=>1,'goodname'=>'戴尔笔记本','price'=>3999, 'num'=>30], ['id'=>2,'goodname'=>'惠普打印机','price'=>1560, 'num'=>10], ['id'=>3,'goodname'=>'戴尔台式电脑','price'=>4580, 'num'=>100] ]; echo json_encode($data); ?>
4.在actions中定义loadingGoods方法,在该方法中使用axios发送异步请求,调用服务器数据------------------
actions:{ loadingGoods(store){ axios.get('./goods.php') .then(response=>{ // 调用mutations中的setGoods方法,传值给 仓库中的mutations,改变state store.commit('setGoods',{goods:response.data}) console.log(response) }) .catch(error=>{ console.log(error) }) } }
5.根实例(根组件)的 生命周期钩子函数mounted 调用 仓库中actions里的 loadingGoods 方法------------------
// 根组件 var vm = new Vue({ el:'#app', data:{ }, store,//把它放在根组件里面,子组件也可以用 components:{ Child }, mounted:function(){ // 根组件,使用dispatch 调用 仓库中actions中的方法 this.$store.dispatch('loadingGoods') } })
预览效果,如下所示:
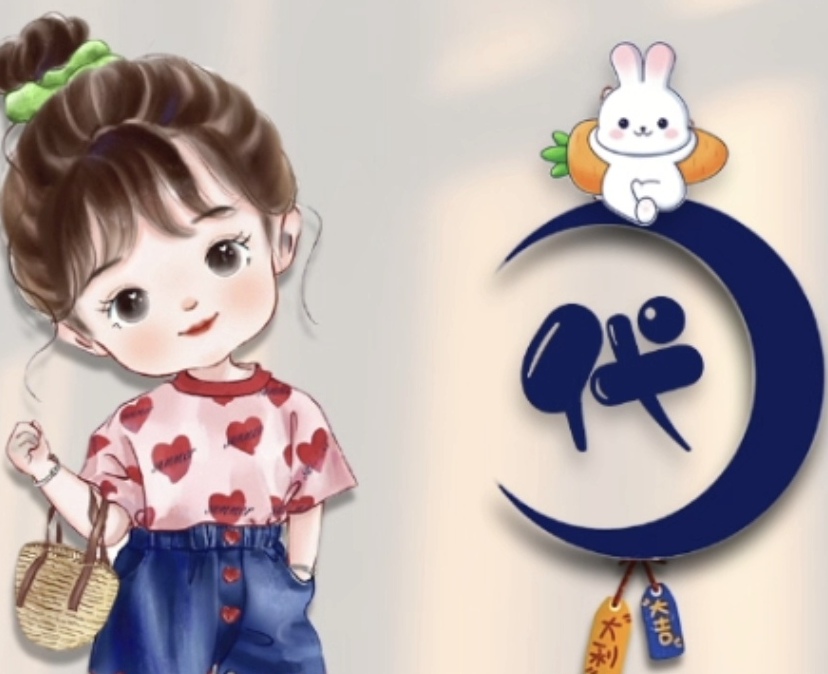